
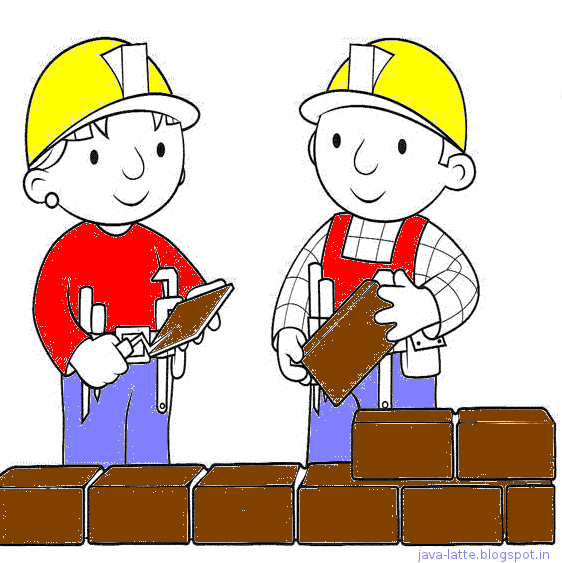
Create a static method with parameter (int age) to do all the checking and return a null if you pass it an illegal value. I can only think of one way to do this. Constructor methodsĬonstructors are really methods. If its illegal, then dont create an object/instance. If a constructor doesn’t take any arguments, it’s considered a “no-argument constructor.” Usually when constructors take arguments, they initialize the new object with certain values.

If a class doesn’t have a constructor, it uses the new keyword in order to create the object. Default constructorsĪll classes rely on constructors in order to create objects. It’s a best practice to always use a constructor with a class even if it doesn’t take an argument. The constructor that runs is determined by the arguments that match the constructor’s parameters. You can have multiple constructors (overloading). However, we often just say “creating an object” or “instantiating the class” instead of using the constructor to create an object. You are actually “constructing” an object using the constructor when you instantiate a class. The constructor for an object is simply the word new before the class name. Even if your class doesn’t have a constructor, the default constructor is made using the new keyword. The syntax for instantiating a class is the same as calling a constructor. Depending on the parameters you’re passing, Java will choose to run the correct constructor. If you pass a string argument when you call the constructor, the M圜onstructor2 will run instead of M圜onstructor1. The constructor that is used is the one whose parameters match the incoming arguments. When there are multiple constructors for the same class (they will aloo have the same name), the arguments specified for each constructor become the distinguishing point.Default constructor is inserted during compilation and hence it will appear only in ‘.class’ file and not in the source code.
Java constructor with only parameters code#
This constructor is inserted by the Java compiler into the class code where there is no constructor implemented by the programmer. It’s a best practice to include a constructor with each class. The default constructor is also called the Empty Constructor.Constructors can take arguments, like methods.Constructors just like a method in its format, except that it has the same name as the class.Constructors have same name as the class.Constructors don’t have a return type (e.g., void).When you create a new object from a constructor, you usually pass in arguments to set values for some of the class’s instance variables.Ĭonstructors have the following characteristics: Constructors use the same name as the class.

best practice is for classes to always have a constructor, even if no argumentsĬonstructors are used to create new objects from classes.a class can have multiple constructors, differentiated by arguments.usually the constructor initializes the object with specific arguments.have same name as class and look just like method.use new keyword: Class object_name = new Class().constructors construct objects from classes.
